Learn how to use a combination of ACF and a PHP code snippet to hide/show WooCommerce payment gateways based on custom logic. In our case we only want specific customers to be able to pay by Purchase Order, but you can use any payment gateway. The purchase order payment gateway comes with our WooCommerce Cart-to-Quote plugin, but there are other PO gateway plugins available if you don’t need all the cart-to-quote functionality.
Set up the ACF field for users/customers
We’re going to add a true/false ACF field to the user profile editor in the backend of WordPress. This will let us enable the PO gateway for individual customers, without needing to put them all into a specific role.
In ACF, create a field group called “Payment Gateway Control” and set up a new true/false field with the name “_can_pay_by_po“:
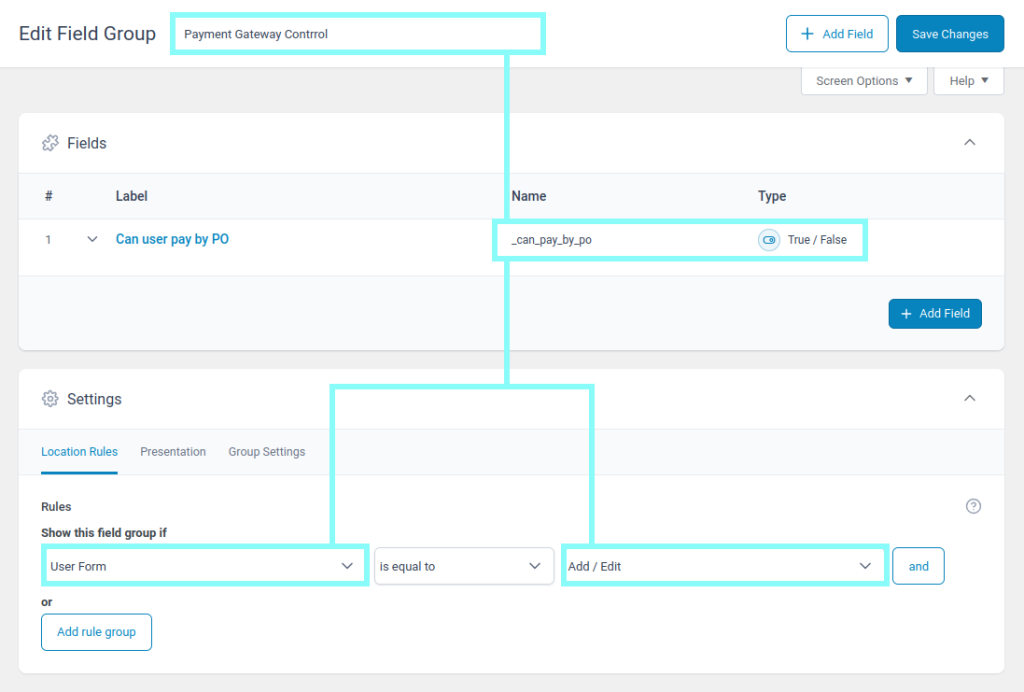
Save the field, then edit your own user account and check it’s in there (note: I used the Stylised UI option in ACF to make it look like a fancy toggle switch).
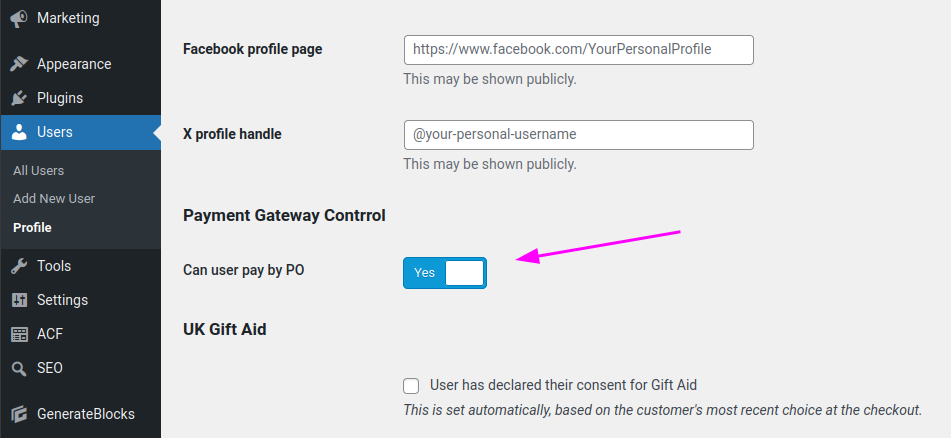
Set up the payment gateway
The payment gateway we want to control should be enabled by default. Our code snippet will disable the gateway if the user is either not logged-in, or if the “Can pay by PO” option is switched off for their account.
Go to your site’s WooCommerce payments settings and enable the gateway. Then right-click on the gateway’s title (in the table) and click on Inspect Element. Look for the TR element and you’ll see the gateway’s slug (id). In our case, the Purchase Order gateway’s slug is “pp-cto-review-purchase-order”.
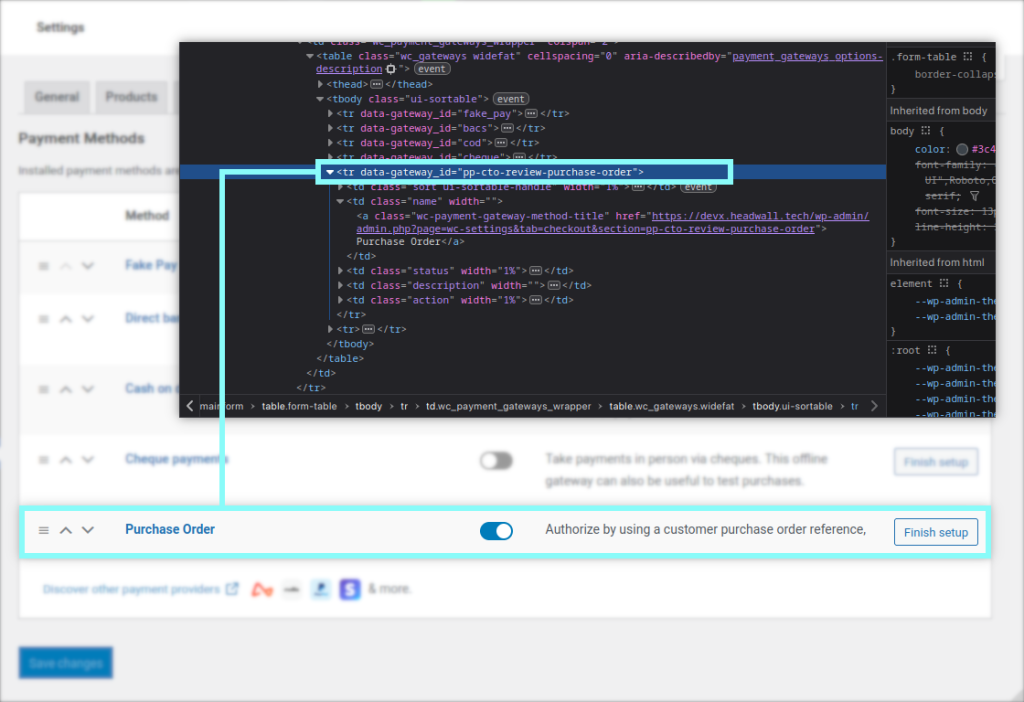
Check that your payment gateway is available in the frontend by putting something in your cart and going to the checkout:
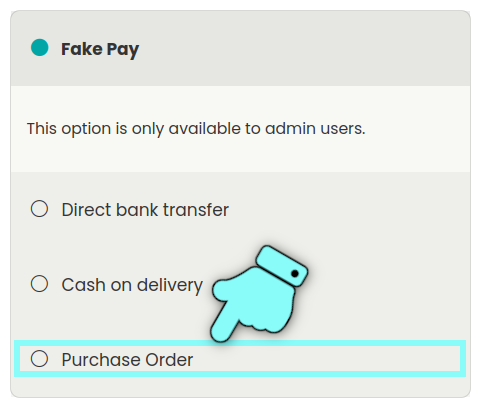
Create the PHP code snippet
The final part of the project is to hook WooCommerce and adjust the available payment gateways on the checkout page. I always create a custom child theme for my projects, so I add PHP snippets in there. But you can use a PHP code snippet manager plugin if you prefer.
Either add the following PHP snippet to your child theme’s functions.php, or set it up in your PHP snippet manager plugin:
/** * Conditionally modify the available payment gateways at the checkout. */ function custom_filter_payment_gateways($gateways) { if (is_admin()) { // Don't change the list of gateways in the back-end. } elseif (!is_checkout()) { // If we're not on the checkout page, don't alter the gateways. } else { // PO is disabled by default $is_purchase_order_enabled = false; // Apply your own logic here and set $is_purchase_order_enabled=false if the // current customer should not have access to the PO payment gateway. if (!is_user_logged_in() || empty(($user_id = get_current_user_id()))) { // Guest users are not allowed to pay by PO here. } elseif (!filter_var(get_user_meta($user_id, '_can_pay_by_po', false), FILTER_VALIDATE_BOOLEAN)) { // The _can_pay_by_po field is not set to true/yes/on } else { $is_purchase_order_enabled = true; } // Remove the PO gateway by its key if (!$is_purchase_order_enabled) { unset($gateways['pp-cto-review-purchase-order']); } } return $gateways; } add_filter('woocommerce_available_payment_gateways', 'custom_filter_payment_gateways', PHP_INT_MAX, 1);
The snippet should be easy enough to read through. Note where we use the get_user_meta() function to get the true/false value of “_can_pay_by_po” for the current logged-in user.
Test the logic
New we can run a few checks to make sure it’s working with the ACF field set to false/off (for your user account), and then when it’s set to true/on:
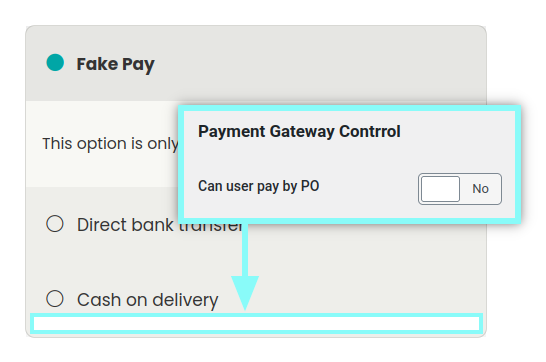
That’s it – just tweak the PHP snippet to suit your store’s business logic requirements.